Typescript to Rust
May 21, 2022 (2 years ago)
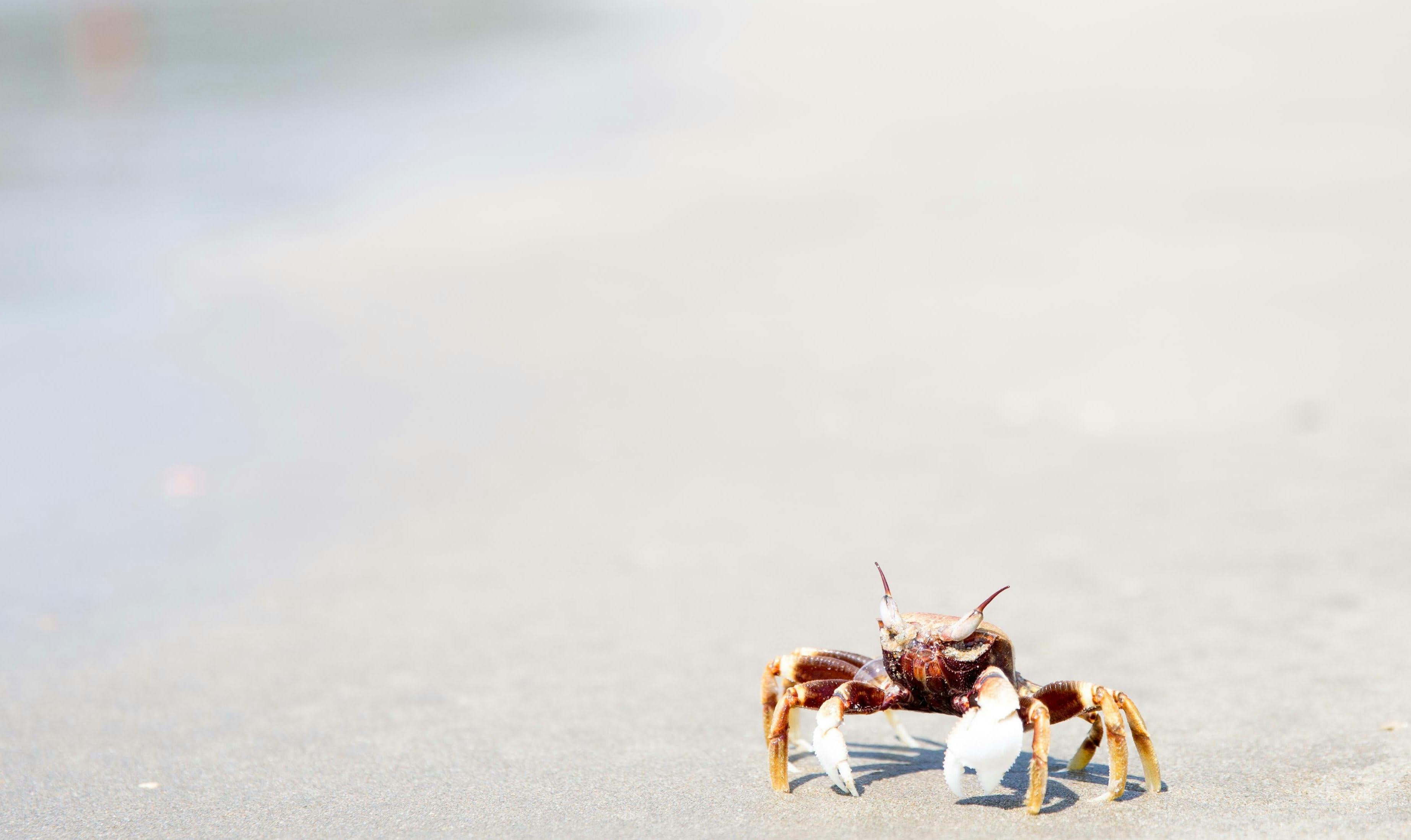
These days, Rust is an extremely popular alternative to traditional js/ts packages. Next.js, the most popular react framework, recently bought up swc
, a Rust compiler. Rust has a stellar community, type safety, and loyal fans — 3 things that C++ does not have.
From the outside perspective, it's weird that the two would have much crossover. Rust is compiled into a binary before it is run, but browsers usually expect uncompiled JS code for execution. In npm packages, node also expects uncompiled JS code. Some npm packages do also rely on compiled system libraries, which was a rude awakening when the (fantastic) M1 mapple computers came out and suddenly node 14’s npm install was poked on packages like sharp.
Packages and web products often push the boundaries of JS scripts in browsers, so there’s a lot of code being compiled for desktop platforms and even web platforms. WebAssembly has allowed Figma to completely smash Sketch (Blockbuster v. Netflix style) by offering both desktop and real-time multiplayer experiences to the browser; which Sketch was not able to remotely match even with a mad dash to Sketch Cloud. On the machine side, swc
is able to smash babel in JS compiling performance. A lot of JS code is being compiled in Rust now — which seems wrong, but I assure you isn’t.
Let's crack open a hello world
in Rust.
fn main() {
println!("Hello World!");
}
As you can see, functions are called with an exclamation mark at the end.
Here's a great tutorial with getting started: https://doc.rust-lang.org/cargo/getting-started/first-steps.html
Data types in Rust
Here’s some key points to help with picking up Rust from typescript:
Rust is very type-safe. There are no ts-ignore comments to save you now! You must type everything you use.
-
objects in Typescript are structs in Rust
interface TypescriptUser { active: boolean; username: string; email: string; }
struct RustUser { active: bool, username: String, email: String }
-
Typescript arrays are Vectors in Rust
let typescript = [1, 2, 3, 4, 5];
let rust = vec![1, 2, 3, 4, 5];
let mut rust = Vec::new(); v.push(1); v.push(2); v.push(3); v.push(4); v.push(5);
-
there is a difference between string literals and strings in Rust.
as_str()
is likeas string
in Ts to help you convert between them
async
/await
is hard in Rust, although you rarely need it
-
cargo-edit gives you cargo add capability, which is almost the same as
npm install
(amazing -
cargo-watch gives you
cargo watch -x run
, which is almost the same as webpack’snpm run start
-
serde-json is how you parse JSON files in Rust.
There are many system libraries in Rust to help you, e.g. git2
.
I am a beginner rustacean, so let me know if I have anything wrong.
Yours in 🦀,
Robbie